38 indexing using labels in dataframe
Multi-level Indexing in Pandas - Python Wife Using a multi-index we create a hierarchy of indices within the data. So, instead of date, we will pass in a list of strings. This will indicate to Pandas that we want all the column names to act as the index for our DataFrame. tech. set_index (['date', 'name'], inplace = True) The resultant DataFrame is a multi-index. pandas.pydata.org › docs › user_guide10 minutes to pandas — pandas 1.5.1 documentation DataFrame.to_numpy() gives a NumPy representation of the underlying data. Note that this can be an expensive operation when your DataFrame has columns with different data types, which comes down to a fundamental difference between pandas and NumPy: NumPy arrays have one dtype for the entire array, while pandas DataFrames have one dtype per column.
DataFrame Indexing: .loc[] vs .iloc[] - Data Science Discovery Indexing Using a Single Label (Row and Column) For the iloc function, to find a specific cell, we can specify the row index (left) and column index (right) separated by a comma. # iloc function # note that the following code uses single brackets ( []). Double brackets ( [ []]) will retrieve rows between the specified integers. df.iloc[0,2] 10.5
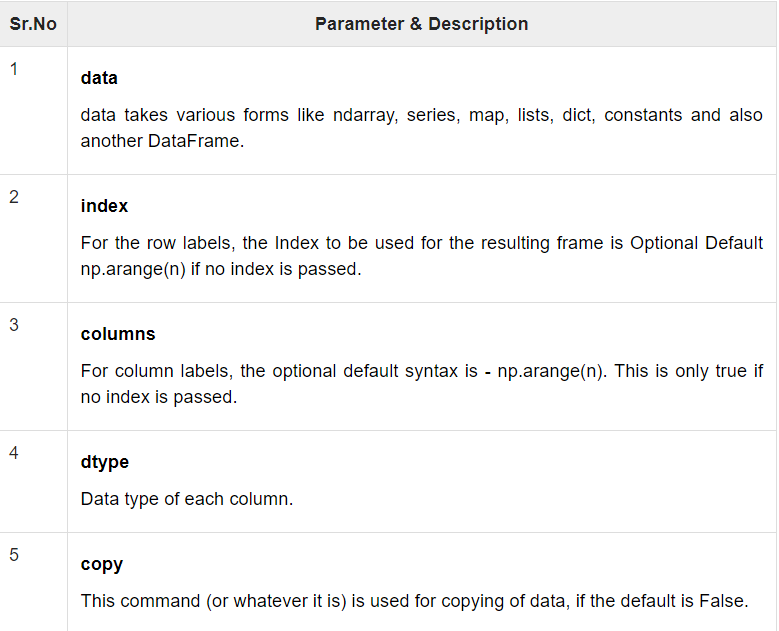
Indexing using labels in dataframe
Python Pandas DataFrame: load, edit, view data | Shane Lynn Rows can also be removed using the “drop” function, by specifying axis=0. Drop() removes rows based on “labels”, rather than numeric indexing. To delete rows based on their numeric position / index, use iloc to reassign the dataframe values, as in the examples below. Pandas DataFrame Indexing Explained: from .loc to .iloc and beyond Indexing can be described as selecting values from specific rows and columns in a dataframe. The row labels (the dataframe index) can be integer or string values, the column labels are usually strings. By indexing, we can be very particular about the selections we make, zooming in on the exact data that we need. Indexing and selecting data — pandas 1.5.1 documentation Indexing and selecting data# The axis labeling information in pandas objects serves many purposes: Identifies data (i.e. provides metadata) using known indicators, important for analysis, visualization, and interactive console display. Enables automatic and explicit data alignment. Allows intuitive getting and setting of subsets of the data set.
Indexing using labels in dataframe. pandas.pydata.org › api › pandaspandas.DataFrame.loc — pandas 1.5.1 documentation pandas.DataFrame.loc# property DataFrame. loc [source] # Access a group of rows and columns by label(s) or a boolean array..loc[] is primarily label based, but may also be used with a boolean array. Allowed inputs are: A single label, e.g. 5 or 'a', (note that 5 is interpreted as a label of the index, and never as an integer position along the ... Series — pandas 1.5.1 documentation Get item from object for given key (ex: DataFrame column). Series.at. Access a single value for a row/column label pair. Series.iat. Access a single value for a row/column pair by integer position. Series.loc. Access a group of rows and columns by label(s) or a boolean array. Series.iloc. Purely integer-location based indexing for selection by ... Get Rows by their Index and Labels - Data Science Parichay You can use the pandas dataframe loc property to access one or more rows of a dataframe by their row labels. The loc property in a pandas dataframe lets you access rows and/or columns using their respective labels. The following is the syntax. # select row with label l df.loc[l] # select rows with labels l, m, and n df.loc[ [l, m, n]] Examples Python Pandas: Get Index Label for a Value in a DataFrame I typically do the following using np.where: import numpy as np idx = df.index [np.where (df ['hair'] == 'blonde')] Which gives the expected result: Index ( [u'mary'], dtype='object') If you want the result in a list, you can use .tolist () method of index Share Follow answered Jun 14, 2017 at 15:28 FLab 6,816 3 34 63 Add a comment Your Answer
pandas DataFrame – Databricks You can choose to specify the axis labels or index that your empty DataFrame will use. If you don’t do this, the pandas DataFrame will automatically construct them for you using common sense rules. ... The indexing that pandas DataFrame lets you do is similar to the kind that you can perform in Excel. The biggest difference is that pandas ... pandas.pydata.org › pandas-docs › stableMultiIndex / advanced indexing — pandas 1.5.1 documentation A MultiIndex can be created from a list of arrays (using MultiIndex.from_arrays()), an array of tuples (using MultiIndex.from_tuples()), a crossed set of iterables (using MultiIndex.from_product()), or a DataFrame (using MultiIndex.from_frame()). The Index constructor will attempt to return a MultiIndex when it is passed a list of tuples. The ... Pandas Dataframe Index in Python - PythonForBeginners.com When a dataframe is created, the rows of the dataframe are assigned indices starting from 0 till the number of rows minus one. However, we can create a custom index for a dataframe using the index attribute. To create a custom index in a pandas dataframe, we will assign a list of index labels to the index attribute of the dataframe. realpython.com › pandas-dataframeThe Pandas DataFrame: Make Working With Data Delightful This Pandas DataFrame looks just like the candidate table above and has the following features: Row labels from 101 to 107; Column labels such as 'name', 'city', 'age', and 'py-score' Data such as candidate names, cities, ages, and Python test scores; This figure shows the labels and data from df:
How to Print Specific Row of Pandas DataFrame - Statology Notice that only the rows located at index positions 3 and 5 are printed. Example 2: Print Row Based on Index Label. The following code shows how to print the row with an index label of 'C' in the DataFrame: #print row with index label 'C' print (df. loc [[' C ']]) points assists rebounds C 19 5 12. Notice that only the row with an index ... Indexing Dataframes. Indexing Dataframes in Pandas | by Vidya Menon ... It is one of the most versatile methods in pandas used to index a dataframe and/or a series method.The loc () function is used to access a group of rows and columns by label (s) or a boolean array. loc [] is primarily label based, but may also be used with a boolean array. The syntax being: Label-based indexing to the Pandas DataFrame - GeeksforGeeks Indexing plays an important role in data frames. Sometimes we need to give a label-based "fancy indexing" to the Pandas Data frame. For this, we have a function in pandas known as pandas.DataFrame.lookup (). The concept of Fancy Indexing is simple which means, we have to pass an array of indices to access multiple array elements at once. pandas.pydata.org › pandas-docs › stableIntro to data structures — pandas 1.5.1 documentation DataFrame.from_dict. DataFrame.from_dict() takes a dict of dicts or a dict of array-like sequences and returns a DataFrame. It operates like the DataFrame constructor except for the orient parameter which is 'columns' by default, but which can be set to 'index' in order to use the dict keys as row labels.
pandas.pydata.org › user_guide › indexingIndexing and selecting data — pandas 1.5.1 documentation Indexing and selecting data# The axis labeling information in pandas objects serves many purposes: Identifies data (i.e. provides metadata) using known indicators, important for analysis, visualization, and interactive console display. Enables automatic and explicit data alignment. Allows intuitive getting and setting of subsets of the data set.
pandas.DataFrame.set_index — pandas 1.5.1 documentation Set the DataFrame index (row labels) using one or more existing columns or arrays (of the correct length). The index can replace the existing index or expand on it. Parameters. keyslabel or array-like or list of labels/arrays. This parameter can be either a single column key, a single array of the same length as the calling DataFrame, or a list ...
The Pandas DataFrame: Make Working With Data Delightful The Pandas DataFrame is a structure that contains two-dimensional data and its corresponding labels.DataFrames are widely used in data science, machine learning, scientific computing, and many other data-intensive fields.. DataFrames are similar to SQL tables or the spreadsheets that you work with in Excel or Calc. In many cases, DataFrames are faster, easier to use, and more …
How To Find Index Of Value In Pandas Dataframe - DevEnum.com 2. df.index.values to Find index of specific Value. To find the indexes of the specific value that match the given condition in Pandas dataframe we will use df ['Subject'] to match the given values and index. values to find an index of matched value. The result shows us that rows 0,1,2 have the value 'Math' in the Subject column.
pandas.DataFrame.loc — pandas 1.5.1 documentation pandas.DataFrame.loc# property DataFrame. loc [source] # Access a group of rows and columns by label(s) or a boolean array..loc[] is primarily label based, but may also be used with a boolean array. Allowed inputs are: A single label, e.g. 5 or 'a', (note that 5 is interpreted as a label of the index, and never as an integer position along the ...
Pandas DataFrame Indexing Streamlined - Table of Contents part of Course 131 Data Munging Tips and Tricks Indexing a Pandas DataFrame for people who don't like to remember things Use loc[] to choose rows and columns by label. Use iloc[] to choose rows and columns by position. Be explicit about both rows and columns, even if it's with ":"
Indexing in Pandas Dataframe using Python | by Kaushik Katari | Towards ... Indexing using .loc method. If we use the .loc method, we have to pass the data using its Label name. Single Row To display a single row from the dataframe, we will mention the row's index name in the .loc method. The whole row information will display like this, Single Row information Multiple Rows
How to drop rows in Pandas DataFrame by index labels? Rows can be removed using index label or column name using this method. Syntax: DataFrame.drop (labels=None, axis=0, index=None, columns=None, level=None, inplace=False, errors='raise') Parameters: labels: String or list of strings referring row or column name. axis: int or string value, 0 'index' for Rows and 1 'columns' for Columns.
MultiIndex / advanced indexing — pandas 1.5.1 documentation A MultiIndex can be created from a list of arrays (using MultiIndex.from_arrays()), an array of tuples (using MultiIndex.from_tuples()), a crossed set of iterables (using MultiIndex.from_product()), or a DataFrame (using MultiIndex.from_frame()). The Index constructor will attempt to return a MultiIndex when it is passed a list of tuples. The ...
MultiIndex / advanced indexing — pandas 1.5.1 documentation You can also construct a MultiIndex from a DataFrame directly, using the method MultiIndex.from_frame(). This is a complementary method to MultiIndex.to_frame(). ... Label-based indexing with integer axis labels is a thorny topic. It has been discussed heavily on mailing lists and among various members of the scientific Python community. In ...
Pandas DataFrame Indexing: Set the Index of a Pandas Dataframe Python list as the index of the DataFrame In this method, we can set the index of the Pandas DataFrame object using the pd.Index (), range (), and set_index () function. First, we will create a Python sequence of numbers using the range () function then pass it to the pd.Index () function which returns the DataFrame index object.
Tutorial: How to Index DataFrames in Pandas - Dataquest Label-based Dataframe Indexing As its name suggests, this approach implies selecting dataframe subsets based on the row and column labels. Let's explore four methods of label-based dataframe indexing: using the indexing operator [], attribute operator ., loc indexer, and at indexer. Using the Indexing Operator
Intro to data structures — pandas 1.5.1 documentation DataFrame.from_dict. DataFrame.from_dict() takes a dict of dicts or a dict of array-like sequences and returns a DataFrame. It operates like the DataFrame constructor except for the orient parameter which is 'columns' by default, but which can be set to 'index' in order to use the dict keys as row labels.
pandas.pydata.org › docs › user_guideMultiIndex / advanced indexing — pandas 1.5.1 documentation A MultiIndex can be created from a list of arrays (using MultiIndex.from_arrays()), an array of tuples (using MultiIndex.from_tuples()), a crossed set of iterables (using MultiIndex.from_product()), or a DataFrame (using MultiIndex.from_frame()). The Index constructor will attempt to return a MultiIndex when it is passed a list of tuples. The ...
10 minutes to pandas — pandas 1.5.1 documentation DataFrame.to_numpy() gives a NumPy representation of the underlying data. Note that this can be an expensive operation when your DataFrame has columns with different data types, which comes down to a fundamental difference between pandas and NumPy: NumPy arrays have one dtype for the entire array, while pandas DataFrames have one dtype per column.When you …
Indexing and selecting data — pandas 1.5.1 documentation Indexing and selecting data# The axis labeling information in pandas objects serves many purposes: Identifies data (i.e. provides metadata) using known indicators, important for analysis, visualization, and interactive console display. Enables automatic and explicit data alignment. Allows intuitive getting and setting of subsets of the data set.
Pandas DataFrame Indexing Explained: from .loc to .iloc and beyond Indexing can be described as selecting values from specific rows and columns in a dataframe. The row labels (the dataframe index) can be integer or string values, the column labels are usually strings. By indexing, we can be very particular about the selections we make, zooming in on the exact data that we need.
Python Pandas DataFrame: load, edit, view data | Shane Lynn Rows can also be removed using the “drop” function, by specifying axis=0. Drop() removes rows based on “labels”, rather than numeric indexing. To delete rows based on their numeric position / index, use iloc to reassign the dataframe values, as in the examples below.
Post a Comment for "38 indexing using labels in dataframe"